Update: 20/8/2023: After the CDK announcement that node 16 is no longer supported after September 2023 we realised that we can’t run CDK and node on Amazon Linux2 for our build agents. We upgraded our agents to AL2023 and found out the native build produces incompatible binaries due to GLIBC upgrades, and Lambda does not support AL2023 runtimes.
We have given up with this native approach due to the fragility of the platform and are investigating AWS Snapstart which now has Java 17 support.
Update: 02/9/2023: We have switched to AWS Snap Start as it appears to be a better trade off for application portability. Short builds and no more binary compatibility issues.
Native Java AWS Lambda refers to Java program that has been compiled down to native instructions so we can get faster “cold start” times on AWS Lambda deployments.
Cold start is the initial time spent in a Lambda Function when it is first deployed by AWS and run up to respond to a request. These cold start times are visible to a caller has higher latency to the first lambda request. Java applications are known for their high cold start times due to the time taken to spin up the Java Virtual Machine and the loading of various java libraries.
We built a small framework that can assemble either a AWS Lambda Java runtime zip, or a provided container implementation of a hello world function. The container provided version is an Amazon Linux 2 Lambda Runtime with a bootstrap shell script that runs our Native Java implementation.
These example lambdas are available (open source) at https://bitbucket.org/limemojito/spring-boot-framework/src/master/development-test/
Note that these timings were against the raw hello java lambda (not the spring cloud function version).
@Slf4j public class MethodHandler { public String handleRequest(String input, Context context) { log.info("Input: " + input); return "Hello World - " + input; } }
Native Java AWS Lambda timings
We open with a “Cold Start” – the time taken to provision the Lambda Function and run the first request. Then a single request to the hot lambda to get the pre-JIT (Just-In-Time compiler) latency. Then ten requests to warm the lambda further so we have some JIT activity. Max Memory use is also shown to get a feel system usage. We run up to 1GB memory sizing to approach 1vCPU as per various discussions online.
Note that we run the lambda at various AWS lambda memory settings as there is a direct proportional link between vCPU allocation and the amount of memory allocated to a lambda (see AWS documentation).
This first set of timings is for a Java 17 Lambda Runtime container running a zip of the hello world function. Times are in milliseconds.
Java Container | 128 | 256 | 512 | 1024 |
Cold Start | 6464 | 5066 | 4054 | 3514 |
1 | 90 | 52 | 16 | 5 |
10X | 60 | 30 | 5 | 4 |
Max Mem | 126 | 152 | 150 | 150 |
Native Java | 128 | 256 | 512 | 1024 |
Cold | 1427 | 1002 | 773 | 670 |
1 | 10 | 4 | 4 | 5 |
10X | 4 | 4 | 3 | 3 |
Max Mem | 111 | 119 | 119 | 119 |
The comparison of the times below show the large performance gains for cold start.
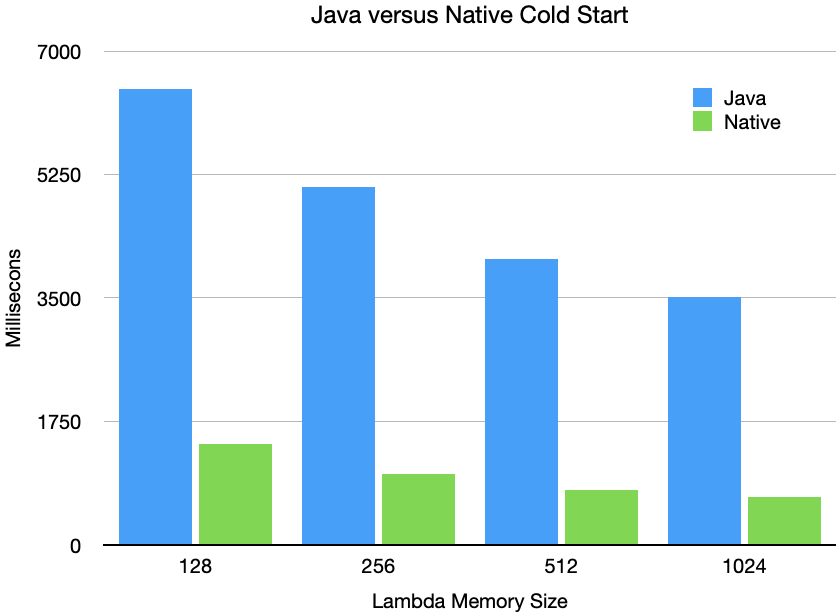
Conclusion
From our results we have a 6X performance improvement in cold starts leading to sub second performance for the initial request.
The native version shows a more consistent warm lambda behaviour due to the native lambda compilation process. Note that the execution times seem to trend for both Java and native down to sub 10ms response times.
While there is a reduction in memory usage this is of no realisable benefit as we configure a larger memory size to get more of a vCPU allocation.
However be aware that build times increased markedly due to the compilation phase (from 2 minutes to 8 for a hello world application). This compilation phase is very CPU and memory intensive so we had to increase our build agents to 6vCPU and 8GB for compiles to work.