After finding Native Java Lambda to be too fragile for runtimes we investigated AWS Snap Start to speed up our cold starts for Java Lambda. While not as fast as native, Snap Start is a supported AWS Runtime mode for Lambda and it is far easier to build and deploy compared to the requirements for native lambda.
How does Snap Start Work?
Snap Start runs up your Java lambda in the initialisation phase, then takes a VM snapshot. That snapshot becomes the starting point for a cold start when the lambda initialises, rather than the startup time of your java application.
With Spring Boot this shows a large decrease in cold start time as the JVM initialisation, reflection and general image setup is happening before the first request is sent.
Snap Start is configured by saving a Version of your lambda. This version phase takes the VM snapshot and loads that instead of the standard java runtime initialisation phase. The runtime required is the offical Amazon Lambda Runtime and no custom images are required.
What are the trade offs for Snap Start?
Version Publishing needs to be added to the lambda deployment. The deployment time is longer as that image needs to be taken when the version is published.
VM shared resources may behave differently to development as they are re-hydrated before use in the cold start case. For example DB connection pools will need to fail and reconnect as they be begin at request time in a disconnected state. However see AWS RDS Proxy for this serverless use case.
As at 26th August 2023 SnapStart is limited to the x86 Architecture for Lambda runtimes.
What are the speed differences?
After warm up there was no difference between a hot JVM and the native compiled hello world program. Cold start however showed a marked difference from memory settings of 512MB and higher due to the proportional allocation of more vCPU.
Times below are in milliseconds.
Architecture | 256 | 512 | 1024 |
Java | 5066 | 4054 | 3514 |
SnapStart | 4689.22 | 2345.2 | 1713.82 |
Native | 1002 | 773 | 670 |
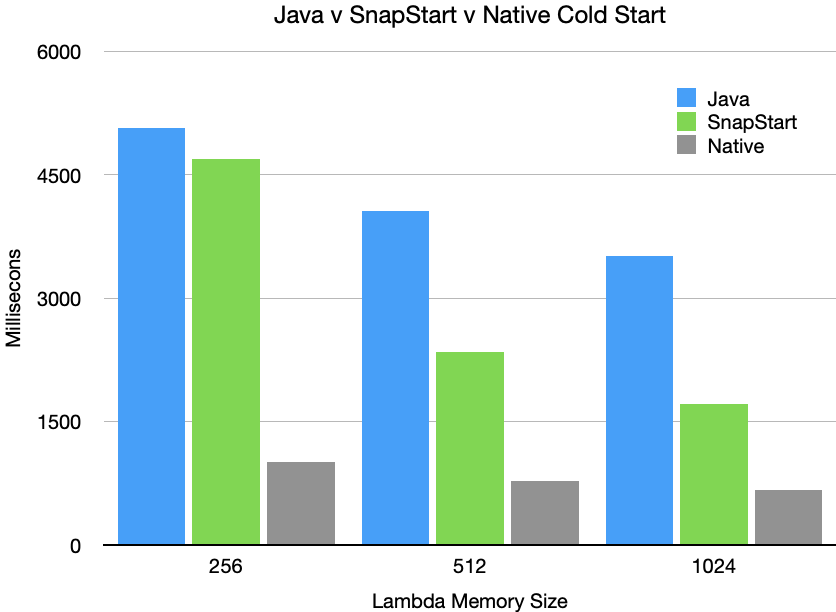
At 1GB with have approximately 1 vCPU for the lambda runtime which makes a significant difference to the cold start times. Memory settings higher than 1vCPU had little effect.
While native is over twice as fast as SnapStart the fragility of deployment for lambda and the massive increase in build times and agent CPU requirements due to compilation was un productive for our use cases.
Snap start adds around 3 minutes to deployments to take the version snapshot (on AWS resources) which we consider acceptable compared to the build agent increase that we needed to do for native (6vCPU and 8GB). As we are back to Java and scripting our agents are back down to 2vCPU and 2GB with build times less than 10 minutes.
How do you integrate Snap Start with AWS CDK?
This is a little tricky as there are not specific CDK Function props to enable SnapStart (as at 26th August 2023). With CDK we have to fall back to a cloud formation primitive to enable snap start and then take a version
Code example from out Open Source Spring Boot framework below.
final IFunction function = new Function(this, LAMBDA_FUNCTION_ID, FunctionProps.builder() .functionName(LAMBDA_FUNCTION_ID) .description("Lambda example with Java 17") .role(role) .timeout(Duration.seconds(timeoutSeconds)) .memorySize(memorySize) .environment(Map.of()) .code(assetCode) .runtime(JAVA_17) .handler(LAMBDA_HANDLER) .logRetention(RetentionDays.ONE_DAY) .architecture(X86_64) .build()); CfnFunction cfnFunction = (CfnFunction) function.getNode().getDefaultChild(); cfnFunction.setSnapStart(CfnFunction.SnapStartProperty.builder() .applyOn("PublishedVersions") .build()); IFunction snapstartVersion = new Version(this, LAMBDA_FUNCTION_ID + "-snap", VersionProps.builder() .lambda(function) .description("Snapstart Version") .build());
In CDK because Version
and Function
both implement IFunction
, you can pass a Version
to route constructs as below.
String apiId = LAMBDA_FUNCTION_ID + "-api"; HttpApi api = new HttpApi(this, apiId, HttpApiProps.builder() .apiName(apiId) .description("Public API for %s".formatted(LAMBDA_FUNCTION_ID)) .build()); HttpLambdaIntegration integration = new HttpLambdaIntegration(LAMBDA_FUNCTION_ID + "-integration", snapstartVersion, HttpLambdaIntegrationProps.builder() .payloadFormatVersion( VERSION_2_0) .build()); HttpRoute build = HttpRoute.Builder.create(this, LAMBDA_FUNCTION_ID + "-route") .routeKey(HttpRouteKey.with("/" + LAMBDA_FUNCTION_ID, HttpMethod.GET)) .httpApi(api) .integration(integration) .build();
Note in the HttpLambdaIntegration
that we pass a Version
rather than the Function
object. This produces the Cloudformation that links the API Gateway integration to your published Snap Start version of the Java Lambda.