We deploy and debug our Java Lambda on development machines using Localstack to emulate and Amazon Web Services (AWS) account. This article walks through the architecture, deployment using our open source java framework to local stack and enabling a debug mode for remote debugging using any Java integrated development environment (IDE).
These capabilities live in our test-utilities module, LambdaSupport.java.
Localstack development architecture
Our build framework uses Docker to deploy a Localstack image, then we use AWS Api calls to deploy a zip of our lambda java classes to the Localstack lambda engine. Due to the size of the zip files, we need to deploy the lambda using a S3 url. We use Localstack’s S3 implementation to emulate the process.
When the lambda is deployed, the Localstack Lambda engine will pull the AWS Lambda Runtime image from public ECR and then perform the deployment steps. Using the Localstack endpoint for lambda we now have a full environment where we can perform a lambda.invoke to test the deployed function.
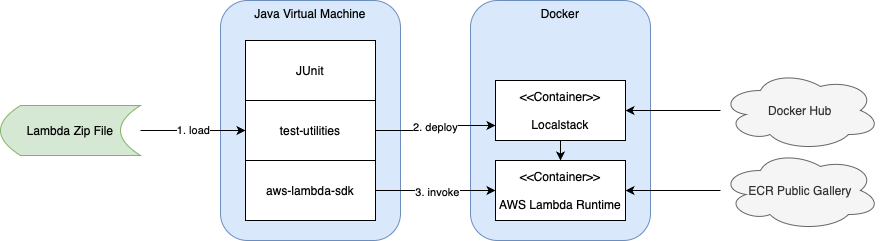
Viewing lambda logs
With the appropriate Localstack configuration we can view lambda logs for both startup and run of the lambda. Note these logs appear in the docker logs for the AWS Lambda Runtime Container. This container spins up when the lambda is deployed.
The easiest method we use to see the logs is to:
- Run the Junit test in debug, with a breakpoint after the lambda invoke.
- When the breakpoint is hit, use
docker ps
anddocker logs
to see the output of the Lambda Runtime. - In IntelliJ Ultimate, you can see the containers deployed via the Services pane after connecting to your docker daemon.
Using the architecture in debug mode
We can use this architecture to remote debug the deployed lambda. Our LambdaSupport class includes configuration on deploy to enable debug mode as per the Localstack documentation https://docs.localstack.cloud/user-guide/lambda-tools/debugging/. With our support class you simply switch from java()
to javaDebug()
and the deploy will configure the runtime for debug mode (port 5050 by default).
In your docker-compose.yml
, set the environment variable LAMBDA_DOCKER_FLAGS=-p 127.0.0.1:5050:5050 -e LS_LOG=debug
.
This enables port passthrough for the java debugger from localhost to port 5050 of the container (assuming that is where the JVM debugging is configured for).
Do not commit this code as it will BLOCK test threads until a debugger is connected (port 5050 by default).
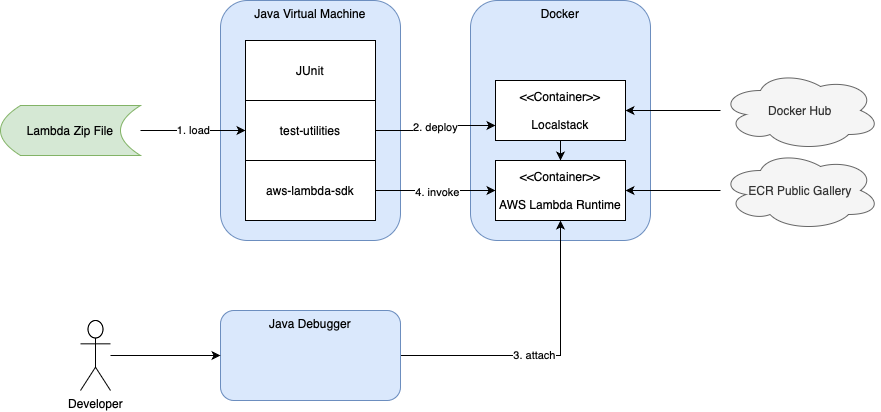
References:
- Lambda ECR images: https://gallery.ecr.aws/lambda/provided
- Lambda ECR image source code: https://github.com/aws/aws-lambda-base-images
- Localstack docker image: https://hub.docker.com/r/localstack/localstack
- Localstack documentation: https://docs.localstack.cloud/overview/
- Localstack Remote Lambda Debugging: https://docs.localstack.cloud/user-guide/lambda-tools/debugging/
Code examples
See https://github.com/LimeMojito/oss-maven-standards/blob/master/development-test/jar-lambda-poc/src/test/java/ApplicationIT.java for a full example.
Adding test-utilities to your maven project
These are included by default if you use our jar-lambda-development parent POM.
See our post about using our build system for maven.
Otherwise you can manually add the support as below (version omitted),
<dependency> <groupId>com.limemojito.oss.test</groupId> <artifactId>test-utilities</artifactId> <scope>test</scope> </dependency> <dependency> <!-- Access for LambdaSupport --> <groupId>software.amazon.awssdk</groupId> <artifactId>lambda</artifactId> <scope>test</scope> </dependency> <dependency> <!-- Access for LambdaSupport --> <groupId>software.amazon.awssdk</groupId> <artifactId>s3</artifactId> <scope>test</scope> </dependency>
Loading the lambda as a static variable in a unit test.
We recommend a static initialised once a junit setup function due to the time to deploy the lambda.
The LambdaSupport.java method performs deployment of the supplied module zip to Localstack S3, then invokes the AWS Lambda API to confirm that the lambda has started cleanly (state == Active).
private static Lambda LAMBDA; ... // environment variables for the lambda configuration final Map<String, String> environment = Map.of( "SPRING_PROFILES_ACTIVE", "integration-test" "SPRING_CLOUD_FUNCTION_DEFINITION","get" ); // using the lambda zip that was built in module ../jar-lambda-poc LAMBDA = lambdaSupport.java("../jar-lambda-poc", LimeAwsLambdaConfiguration.LAMBDA_HANDLER, environment);
Invoking the lambda for black box testing
This example is using a static variable for the Lambda, JUnit 5 and assert4J. An AWS API Gateway event JSON is loaded and invoked to the deployed lambda. The result is asserted.
Full example is in our oss-maven-standards repository as in integration test (IT, run by failsafe).
@Test public void shouldCallTransactionPostOkApiGatewayEvent() { final APIGatewayV2HTTPEvent event = json.loadLambdaEvent("/events/postApiEvent.json", APIGatewayV2HTTPEvent.class); final APIGatewayV2HTTPResponse response = lambdaSupport.invokeLambdaEvent(LAMBDA, event, APIGatewayV2HTTPResponse.class); assertThat(response.getStatusCode()).isEqualTo(200); String output = json.parse(response.getBody(), String.class); assertThat(output).isEqualTo("world"); }
Localstack lambda deployment debug example
We alter the setup to use the deprecated javaDebug function. Do not commit this code as it will BLOCK test threads until a debugger is connected (port 5050 by default).
For a clean setup in Intelij that waits for the lambda to start in debug mode, see the excellent article on Localstack https://docs.localstack.cloud/user-guide/lambda-tools/debugging/ “Configuring IntelliJ IDEA for remote JVM debugging”.
// using the lambda zip that was built in module ../jar-lambda-poc LAMBDA = lambdaSupport.javaDebug("../jar-lambda-poc", LimeAwsLambdaConfiguration.LAMBDA_HANDLER, environment);